Introduction
Business Process Model and Notation (BPMN) is a graphical representation for specifying business processes in a business process model. Camunda is a powerful open-source platform for workflow and business process automation. In this blog post, we will guide you through creating a simple BPMN workflow in Camunda and demonstrate how to access and interact with this workflow from a Java application using the Spring Boot framework.
Creating a Simple BPMN Workflow in Camunda
Step 1: Setting Up Camunda Modeler
First, download and install the Camunda Modeler from the official website. The Camunda Modeler allows you to create BPMN diagrams, deploy them to the Camunda engine, and test them.
Step 2: Designing the BPMN Workflow
- Open Camunda Modeler: Open the Camunda Modeler and create a new BPMN diagram.
- Create a Start Event: Drag and drop a Start Event onto the canvas.
- Create a User Task: Drag and drop a User Task onto the canvas and connect it to the Start Event.
- Create an End Event: Drag and drop an End Event onto the canvas and connect it to the User Task.
Your BPMN diagram should look like this:
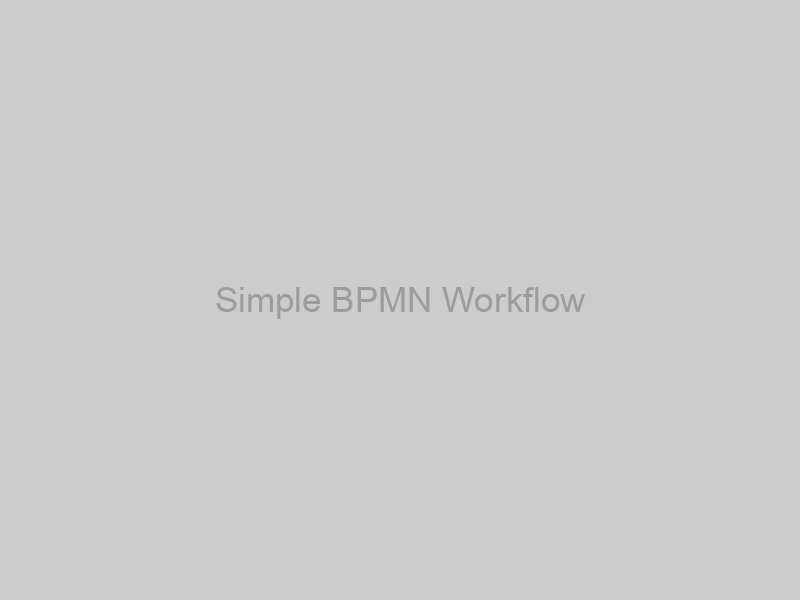
- Configure the User Task: Click on the User Task and set the
Id
touserTask1
and theName
toApprove Request
. - Save the Diagram: Save the diagram with a meaningful name, for example,
simple-workflow.bpmn
.
Step 3: Deploying the Workflow to Camunda
To deploy the BPMN file to Camunda, you need a running Camunda engine. You can either set up Camunda on your local machine or use a pre-configured Docker image.
Deploying Using Camunda Spring Boot Starter
- Create a Spring Boot Project: Use Spring Initializr to create a new Spring Boot project with the following dependencies:
- Spring Web
- Spring Data JPA
- H2 Database
- Camunda BPM Spring Boot Starter
- Add Camunda Dependencies:
<dependency>
<groupId>org.camunda.bpm.springboot</groupId>
<artifactId>camunda-bpm-spring-boot-starter</artifactId>
<version>7.17.0</version>
</dependency>
- Application Properties:
spring.datasource.url=jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.h2.console.enabled=true
camunda.bpm.generate-unique-process-engine-name=true
camunda.bpm.process-engine-name=default
- Place the BPMN File: Put the
simple-workflow.bpmn
file in thesrc/main/resources
directory of your Spring Boot project. - Main Application Class:
package com.example.workflow;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class WorkflowApplication {
public static void main(String[] args) {
SpringApplication.run(WorkflowApplication.class, args);
}
}
- Run the Application: Start the Spring Boot application, which will deploy the BPMN file to the Camunda engine automatically.
Accessing the BPMN Workflow from Java Using Spring Boot
Step 1: Starting the Process Instance
To start a process instance of the deployed BPMN workflow, you need to interact with the Camunda engine API.
- Create a REST Controller:
package com.example.workflow.controller;
import org.camunda.bpm.engine.RuntimeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class WorkflowController {
@Autowired
private RuntimeService runtimeService;
@PostMapping("/start-process")
public String startProcess() {
runtimeService.startProcessInstanceByKey("simpleWorkflow");
return "Process started successfully!";
}
}
Step 2: Querying Tasks
To query and complete user tasks in the workflow, you need to use the TaskService API.
- Add Task Query and Completion Logic:
package com.example.workflow.controller;
import org.camunda.bpm.engine.TaskService;
import org.camunda.bpm.engine.task.Task;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class TaskController {
@Autowired
private TaskService taskService;
@GetMapping("/tasks")
public List<Task> getTasks() {
return taskService.createTaskQuery().list();
}
@PostMapping("/complete-task")
public String completeTask(String taskId) {
taskService.complete(taskId);
return "Task completed successfully!";
}
}
Step 3: Running the Application and Testing
- Start the Application: Run the Spring Boot application.
- Start a Process Instance: Use a tool like Postman or curl to send a POST request to
http://localhost:8080/start-process
. - Query Tasks: Send a GET request to
http://localhost:8080/tasks
to retrieve the list of tasks. - Complete a Task: Send a POST request to
http://localhost:8080/complete-task
with thetaskId
as a parameter to complete a user task.
Summary
In this blog post, we have demonstrated how to create a simple BPMN workflow using the Camunda Modeler and how to deploy it to a Camunda engine running in a Spring Boot application. We also covered how to interact with the workflow from a Java application, including starting process instances, querying tasks, and completing tasks using the Camunda engine’s APIs.
By following these steps, you can leverage the powerful capabilities of Camunda to automate your business processes and integrate them seamlessly into your Spring Boot applications. Camunda provides a robust platform for workflow automation that can help streamline your business operations and improve efficiency.